📘 قراءة كتاب ES6 tutorialspoint أونلاين


حول البرنامج التعليمي
ECMAScript (ES) هو أحد مواصفات لغة البرمجة النصية الموحدة من قبل ECMAScript
International. يتم استخدامه بواسطة التطبيقات لتمكين البرمجة النصية من جانب العميل.
تخضع لغات مثل JavaScript و Jscript و ActionScript لهذه المواصفات.
يقدم لك هذا البرنامج التعليمي كيفية تنفيذ ES6 في JavaScript.
الجمهور
تم إعداد هذا البرنامج التعليمي لمطوري JavaScript الذين يحرصون على معرفة
الفرق بين ECMAScript 5 و ECMAScript 6. وهو مفيد لأولئك الذين يرغبون في
تعلم أحدث التطورات في اللغة وتنفيذها في JavaScript.
المتطلبات الأساسية
يجب أن يكون لديك فهم أساسي لـ JavaScript لتحقيق أقصى استفادة من هذا البرنامج التعليمي.
إخلاء المسؤولية وحقوق الطبع والنشر
حقوق الطبع والنشر لعام 2016 بواسطة Tutorials Point (I) Pvt. المحدودة
جميع المحتوى والرسومات المنشورة في هذا الكتاب الإلكتروني هي ملك دروس نقطة (I)
الجندي. Ltd. يُحظر على مستخدم هذا الكتاب الإلكتروني إعادة استخدام أو الاحتفاظ أو نسخ أو توزيع أو إعادة نشر
أي محتويات أو جزء من محتويات هذا الكتاب الإلكتروني بأي شكل من الأشكال دون موافقة خطية
من الناشر.
نحن نسعى جاهدين لتحديث محتويات موقعنا على الويب والبرامج التعليمية في الوقت المناسب
وبأكبر قدر ممكن من الدقة ، ومع ذلك ، قد تحتوي المحتويات على معلومات غير دقيقة أو أخطاء. نقطة دروس (I) الجندي.
لا تقدم أي ضمانات فيما يتعلق بدقة أو توقيت أو اكتمال موقعنا
أو محتوياته بما في ذلك هذا البرنامج التعليمي. إذا اكتشفت أي أخطاء على موقعنا أو
في هذا البرنامج التعليمي ، يرجى إعلامنا على [email protected].
ES6
ii
جدول المحتويات
حول البرنامج التعليمي .......................................... .................................................. ........................................ i
الجمهور ........ .................................................. .................................................. ...................................... i
المتطلبات الأساسية .......... .................................................. .................................................. .............................. i
إخلاء المسؤولية وحقوق النشر ................ .................................................. .................................................. ......... أنا
جدول المحتويات ............................................... .................................................. ................................... ii
1. ES6 - نظرة عامة ......... .................................................. .................................................. .... 1
جافا سكريبت ............................................ .................................................. .................................................. 1
2. ES6 - البيئة ............................................ .................................................. ............ 2
إعداد البيئة المحلية .................................. .................................................. ..................................... 2
التثبيت على Windows ............................................... .................................................. ........................ 2
التثبيت على نظام التشغيل Mac OS X .................... .................................................. .................................................. .3
التثبيت على Linux ............................................. .................................................. ................................. 4
دعم بيئة التطوير المتكاملة (IDE) ......... .................................................. ................... 4
كود Visual Studio ... .................................................. .................................................. ... 4
اقواس ................................................. .................................................. ............................................... 7
3. ES6 - SYNTAX .................................................. .................................................. ................... 10
المسافة البيضاء وفواصل الأسطر .......................... .................................................. ...................................... 11
تعليقات في JavaScript ........ .................................................. .................................................. ............ 11
كود JavaScript الأول الخاص بك ................................. .................................................. .................................. 12
تنفيذ المدونة ............................................... .................................................. ............................... 12
Node.js و JS / ES6 ........... .................................................. .................................................. ................. 13
الوضع الصارم ............................. .................................................. .................................................. .... 13
ES6 والرفع .......................................... .................................................. ........................................ 14
ES6
iii
4. ES6 - المتغيرات .. .................................................. .................................................. ......... 15
اكتب النحو ................................................ .................................................. ......................................... 15
JavaScript والطباعة الديناميكية ... .................................................. .................................................. ..... 16
JavaScriptVariable Scope .................................................. .................................................. ........................... 16
نطاق السماح والمنع ... .................................................. .................................................. ... 17
التأسيس ............................................ .................................................. ................................................. 18
ES6 والرفع المتغير .............................................. .................................................. ...................... 18
5. ES6 - المشغلون ...................... .................................................. ..................................... 20
عوامل حسابية .......... .................................................. .................................................. .............. 20
العوامل العلائقية ................................. .................................................. .......................................... 22
العوامل المنطقية ..... .................................................. .................................................. ......................... 23
عوامل التشغيل على مستوى البت ................................................ .................................................. ............................... 24
مشغلي التخصيص ................ .................................................. .................................................. ... 26
مشغلون متنوعون ........................................ .................................................. ............................ 27
نوع مشغلي ................... .................................................. .................................................. .............. 29
6. ES6 - اتخاذ القرار ............................. .................................................. .................... 30
بيان if ............................................... .................................................. ................................... 30
بيان if ... else ......... .................................................. .................................................. .............. 32
الآخر ... إذا سلم .............................. .................................................. ................................................ 33
و التبديل ... بيان الحالة .............................................. .................................................. .................. 34
7. ES6 - LOOPS .......................... .................................................. .......................................... 38
حلقة محددة ................................................ .................................................. ....................................... 38
حلقة غير محددة ........ .................................................. .................................................. .......................... 41
بيانات التحكم في الحلقة ... .................................................. ........................................... 44
استخدام الملصقات للتحكم في التدفق .................................................. .................................................. ....... 45
ES6
iv
8. ES6 - الوظائف ................................... .................................................. ......................... 49
تصنيف الوظائف ............................................... .................................................. .................... 49
معلمات الراحة ........................... .................................................. .................................................. ..... 52
وظيفة مجهولة .......................................... .................................................. ................................ 52
منشئ الوظيفة .............. .................................................. .................................................. .... 53
وظائف العودية وجافا سكريبت ......................................... .................................................. ............ 54
وظائف لامدا ................................................ .................................................. ............................... 55
التعبير عن الوظيفة وإعلان الوظيفة ... .................................................. ....................... 56
وظيفة الرفع ........................ .................................................. .................................................. ...... 57
التعبير عن الوظيفة الذي تم استدعاؤه فورًا ... .................................................. ... 57
وظائف المولد ............................................ .................................................. ............................... 58
9. ES6 - الأحداث ............................................. .................................................. ..................... 61
مناولي الأحداث .......................... .................................................. .................................................. ... 61
onclick نوع الحدث ...................................... .................................................. ......................................... 61
عند تقديم نوع الحدث ...... .................................................. .................................................. ................... 62
onmouseover and onmouseout ........................... .................................................. ............................... 63
أحداث HTML 5 القياسية .............................................. .................................................. ....................... 63
10. ES6 - ملفات تعريف الارتباط ..................... .................................................. ............................................ 70
كيف يعمل ؟. .................................................. .................................................. .................................. 70
تخزين ملفات تعريف الارتباط ............. .................................................. .................................................. .................... 70
قراءة ملفات تعريف الارتباط ........................... .................................................. .................................................. ..... 72
تعيين تاريخ انتهاء صلاحية ملفات تعريف الارتباط .............................................. .................................................. ................... 73
حذف ملف تعريف الارتباط ........................... .................................................. .................................................. ... 74
11. ES6 - تعديل الصفحة ........................................ .................................................. .............. 76
إعادة توجيه صفحة JavaScript ................................ .................................................. ................................ 76
إعادة التوجيه وتحسين محركات البحث ............ .................................................. ........................... 77
ES6
سنة النشر : 2016م / 1437هـ .
حجم الكتاب عند التحميل : 2.5 ميجا بايت .
نوع الكتاب : pdf.
عداد القراءة:
اذا اعجبك الكتاب فضلاً اضغط على أعجبني و يمكنك تحميله من هنا:
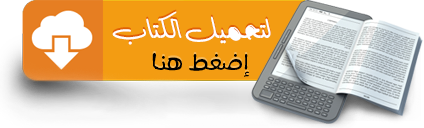
شكرًا لمساهمتكم
شكراً لمساهمتكم معنا في الإرتقاء بمستوى المكتبة ، يمكنكم االتبليغ عن اخطاء او سوء اختيار للكتب وتصنيفها ومحتواها ، أو كتاب يُمنع نشره ، او محمي بحقوق طبع ونشر ، فضلاً قم بالتبليغ عن الكتاب المُخالف:
قبل تحميل الكتاب ..
يجب ان يتوفر لديكم برنامج تشغيل وقراءة ملفات pdf
يمكن تحميلة من هنا 'http://get.adobe.com/reader/'